UILabel을 추가하면 간단하게 글자를 넣을 수 있다.
하지만 sub view가 많아지면 어플이 무거워 질 수 있는데 이럴때에는 NSString을 써주면 좋다.
그렇다면 NSString를 UILabel처럼 배경색을 넣어서 마치 view인듯 사용해 보자~
먼저, NSString이 들어있는 클래스를 하나 만들자! NSString를 상속받아 확장해도 좋다. 나는 그냥 했다. TextBox라고 명명하겠다.
class TextBox {
//정렬키 상수
static let CENTER = 0
static let LEFT = 8
static let RIGHT = 16
static let TOP = 1
static let BOTTOM = 2
var mText: NSString
var mAlign: Int
var mFontAttrDic: [String: NSObject]
var mRect, mBgRect: CGRect
var mPadding: (left: CGFloat, top: CGFloat, right: CGFloat, bottom: CGFloat)
var mBgColor: UIColor
var mCornerRadius: CGFloat
init(text: NSString, align: Int, padding: (left: CGFloat, top: CGFloat, right: CGFloat, bottom: CGFloat), font: UIFont, color: UIColor, bgColor: UIColor, corner: CGFloat = 0) {
mText = text
mAlign = align //정렬
mFontAttrDic = [
NSForegroundColorAttributeName: color,
NSParagraphStyleAttributeName: NSMutableParagraphStyle(),
NSFontAttributeName: font
]
mRect = CGRect() //글자 위치크기
mBgRect = CGRect() //배경 위치크기
mPadding = padding //글자와배경 간격
mBgColor = bgColor //배경색
mCornerRadius = corner //둥근사각형 각크기
}
func setPoint(x: CGFloat, _ y: CGFloat) -> TextBox {
//글자 원본크기
let size = mText.sizeWithAttributes(mFontAttrDic)
let width = size.width
let height = size.height
//크기 설정
let padding = mPadding
mRect.size.width = width
mRect.size.height = height
mBgRect.size.width = width + padding.left + padding.right
mBgRect.size.height = height + padding.top + padding.bottom
//위치 설정
//가로 정렬
switch mAlign >> 3 {
case 1: //왼쪽 정렬
mRect.origin.x = x + padding.left
mBgRect.origin.x = x
case 2: //오른쪽 정렬
let x = x - padding.right - width
mRect.origin.x = x
mBgRect.origin.x = x - padding.left
default: //가운데 정렬
let x = x - (width / 2)
mRect.origin.x = x
mBgRect.origin.x = x - padding.left
}
//세로 정렬
switch mAlign & 7 {
case 1: //위쪽 정렬
mRect.origin.y = y + padding.top
mBgRect.origin.y = y
case 2: //아래쪽 정렬
let y = y - padding.bottom - height
mRect.origin.x = y
mBgRect.origin.x = y - padding.top
default: //가운데 정렬
let y = y - (height / 2)
mRect.origin.y = y
mBgRect.origin.y = y - padding.top
}
return self
}
func draw(context: CGContext) {
//배경 출력
CGContextSetFillColorWithColor(context, mBgColor.CGColor)
if mCornerRadius == 0 {
//사각형
CGContextFillRect(context, mBgRect)
}
else {
//둥근사각형
CGContextAddPath(context, UIBezierPath(roundedRect: mBgRect, byRoundingCorners: .AllCorners, cornerRadii: CGSizeMake(mCornerRadius, mCornerRadius)).CGPath)
CGContextFillPath(context)
}
//글자 출력
mText.drawInRect(mRect, withAttributes: mFontAttrDic)
}
}
위의 소스를 보면 알겠지만 미리 색이나 글자배경 간격등을 정해준 후 setPoint로 위치를 찍어주면 글자크기를 얻어 배경을 설정값에 맞게 만들어주고 draw로 그려준다.
자.. UIView를 확장하여 위의 TextBox를 이용해 보자
class TextBoxsView: UIView {
var mTextBoxs: [TextBox]!
func initTextBoxs() {
//폰트
let font = UIFont.systemFontOfSize(15)
mTextBoxs = [TextBox]()
//사각형 배경
mTextBoxs.append(TextBox(text: "Rect", align: TextBox.CENTER, padding: (30, 50, 40, 10), font: font, color: UIColor.whiteColor(), bgColor: UIColor.blackColor()).setPoint(100, 200))
//둥근사각형 배경
mTextBoxs.append(TextBox(text: "RoundRect", align: TextBox.LEFT, padding: (30, 30, 30, 30), font: font, color: UIColor.greenColor(), bgColor: UIColor.blueColor(), corner: 10).setPoint(100, 400))
}
override func drawRect(rect: CGRect) {
let context = UIGraphicsGetCurrentContext()
//출력
for textBox in mTextBoxs {
textBox.draw(context!)
}
}
}
테스트를 위해 설정값이 틀린 사각형과 둥근사각형 둘을 만들었다. initTextBoxs를 호출하면 된다.
끝으로 ViewController에서 view를 위의 TextBoxsView로 바꾸고 호출한다.
class TextBoxViewController: ViewController {
override func viewDidLoad() {
super.viewDidLoad()
(view as! TextBoxsView).initTextBoxs()
}
}
결과♡
도움이 되셨다면~ 정성으로 빚은 저희 앱! 많은 이용 바래요:)
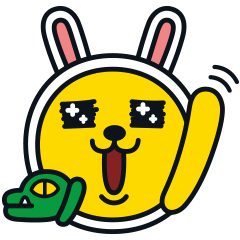
https://meorimal.com/index.html?tab=spaceship
우주선 - 방치형 인공지능 투자 체험기
미리 맛보는 인공지능 투자!
(주)머리말 meorimal.com
https://meorimal.com/subway.html
지하철어디있니
더이상 고민하지 마세요. 뛸지 말지 딱 보면 알죠.
(주)머리말 meorimal.com
'개발 > ios' 카테고리의 다른 글
손쉽게 아이폰 앱을 새로 실행 시키는 코드 한줄 (1) | 2018.03.26 |
---|---|
UIView의 원 테두리가 얇거나 작아보일때... (0) | 2018.01.29 |
UIButton 이미지를 손쉽게 정렬하자~ (0) | 2017.05.30 |
UIView에 동적으로 버튼을 넣을때 크기를 같게 맞출려면? (0) | 2017.04.25 |
기준점을 중앙으로 Scale UIView 애니메이션 하기 (0) | 2017.03.21 |